Spring Bootでコントローラ(Controller)からビュー(View)に値を返却して画面に表示させたい場合、以下4つの方法があります。4つのどの方法でも同じ結果となります。
- Model
- Map
- ModelMap
- ModelAndView
どの方法を採用するかはプロジェクトのコーディング規約に従うのが良いと思いますが、私としてはModelAndViewがおすすめです。
Controller(コントローラ)
@GetMappingでURLルーティングを指定しています。実際に動かして試す時はそれぞれ@GetMappingで指定したURLにブラウザでアクセスします。最後に動作確認結果を載せてあります。
package com.example.learn.controllers;
import java.util.Map;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class TopController {
@GetMapping("/")
public String view(Model model) {
model.addAttribute("name", "yamada taro");
model.addAttribute("address", "japan");
return "top";
}
@GetMapping("/map")
public String viewModelMap(Map model) {
model.put("name", "yamada taro(Map)");
model.put("address", "japan");
return "top";
}
@GetMapping("/modelmap")
public String viewModelMap(ModelMap model) {
model.addAttribute("name", "yamada taro(ModelMap)");
model.addAttribute("address", "japan");
return "top";
}
@GetMapping("/modelandview")
public ModelAndView viewModelAndView() {
ModelAndView model = new ModelAndView();
model.addObject("name", "yamada taro(ModelAndView)");
model.addObject("address", "japan");
model.setViewName("top");
return model;
}
}
ModelAndViewはそのクラス名の通り、返却値とビューの情報を持ちますので、このクラスに両方を設定して返却する形になります。
View(ビュー)
以下のビューで動作確認を行いました。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>DEMO</title>
</head>
<body>
<h1>TOP PAGE</h1>
①要素値
[[ ${name} ]]
<br>
②属性値
<span th:text="${address}"></span>
<br><button onclick="location.href='/'">@GetMapping("/")を表示</button>
<br><button onclick="location.href='/map'">@GetMapping("/map")を表示</button>
<br><button onclick="location.href='/modelmap'">@GetMapping("/modelmap")</button>
<br><button onclick="location.href='/modelandview'">@GetMapping("/modelandview")</button>
</body>
</html>
動作確認
遷移先の画面ごとに要素値、属性値が切り替わることを確認できます。
http://localhost:8080 に接続
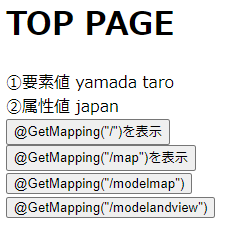
http://localhost:8080/map に接続
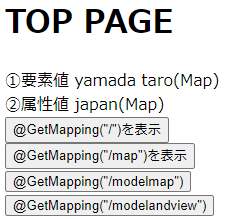
http://localhost:8080/modelmap に接続
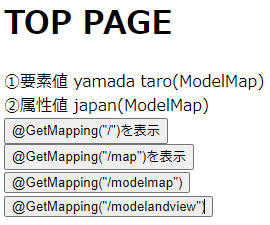
http://localhost:8080/modelandview に接続
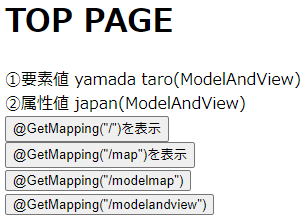
コメント