SQLだけでSELECT結果をグルーピングして階層で取得することはできません。MyBatisの「collection」を使用すると、DBからの取得結果を親子関係の階層で取得することができます。つまり、SELECTしただけで、配列が階層になってくれているので、あらためて、取得結果をループして、クラスに詰めなおすといった作業が不要になります。
パターン①SQLだけのSELECT結果
同じ親(従業員)に対し、子(販売実績)がセットになった1レコードとして取得します。いわゆる一般的なSELECT結果です。
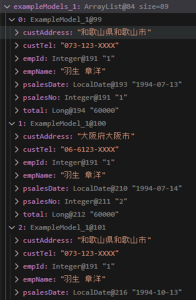
パターン②MyBatisの「collection」を使用したSELECT結果
同じ親(従業員)に対し、子(販売実績)が親子関係の階層で取得します。この時点で階層化されているので、ビジネスロジックで処理しやすい形で取得することができます。
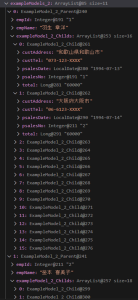
ExampleMapper.xml
パターン①②のどちらもSQLは同じで、resultMapの記載が異なります。
<mapper namespace="your_space">
<!-- パターン①SQLだけのSELECT結果 -->
<resultMap id="resultMap_1" type="com.jpterrace.learning.model.learningdb.ExampleModel_1">
<id column="emp_id" property="empId" />
<result column="emp_name" property="empName" />
<result column="psales_no" property="psalesNo" />
<result column="psales_date" property="psalesDate" />
<result column="cust_address" property="custAddress" />
<result column="cust_tel" property="custTel" />
<result column="total" property="total" />
</resultMap>
<select id="selectExample_1" resultMap="resultMap_1">
<!-- 従業員ごとの販売実績を取得する -->
SELECT
employee.emp_id,
employee.emp_name,
packedsales.psales_no,
packedsales.psales_date,
packedsales.cust_address,
packedsales.cust_tel,
packedsales.total
FROM
employee
LEFT JOIN packedsales
ON employee.emp_id = packedsales.emp_id
ORDER BY emp_id, psales_no
</select>
<!-- パターン②MyBatisの「collection」を使用したSELECT結果 -->
<resultMap id="resultMap_2" type="your_pace.ExampleModel_2_Parent">
<result column="emp_id" property="empId" />
<result column="emp_name" property="empName" />
<collection property="exampleModel_2_Childs" ofType="your_pace.ExampleModel_2_Child">
<result column="psales_no" property="psalesNo" />
<result column="psales_date" property="psalesDate" />
<result column="cust_address" property="custAddress" />
<result column="cust_tel" property="custTel" />
<result column="total" property="total" />
</collection>
</resultMap>
<select id="selectExample_2" resultMap="resultMap_2">
<!-- 従業員ごとの販売実績を取得する -->
SELECT
employee.emp_id,
employee.emp_name,
packedsales.psales_no,
packedsales.psales_date,
packedsales.cust_address,
packedsales.cust_tel,
packedsales.total
FROM
employee
LEFT JOIN packedsales
ON employee.emp_id = packedsales.emp_id
ORDER BY emp_id, psales_no
</select>
ExampleMapper.java
public interface ExampleMapper {
List<ExampleModel_1> selectExample_1();
List<ExampleModel_2_Parent> selectExample_2();
}
ExampleModel_1.java
public class ExampleModel_1 {
private Integer empId;
private String empName;
private Integer psalesNo;
private LocalDate psalesDate;
private String custAddress;
private String custTel;
private Long total;
}
ExampleModel_2_Parent.java
public class ExampleModel_2_Parent {
private Integer empId;
private String empName;
List<ExampleModel_2_Child> exampleModel_2_Childs;
}
ExampleModel_2_Child.java
public class ExampleModel_2_Child {
private Integer psalesNo;
private LocalDate psalesDate;
private String custAddress;
private String custTel;
private Long total;
}
コメント